Merge Two Sorted Lists Algorithm
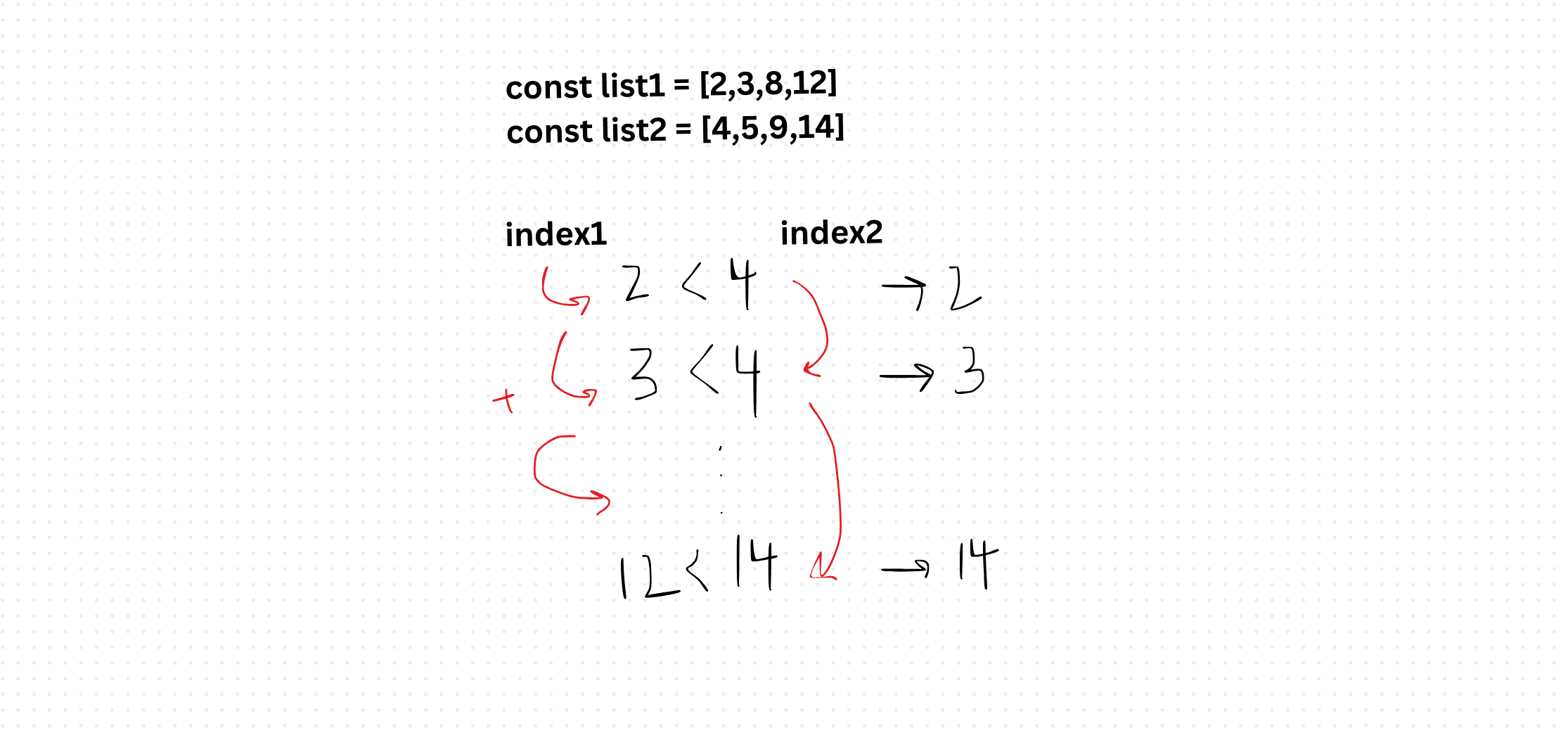
At its core, the Merge Two Sorted Lists algorithm aims to combine two sorted linked lists into a single sorted linked list. Given two input lists, the algorithm traverses both lists simultaneously, comparing the values of nodes at each position and merging them into a new sorted list.
The Merge Two Sorted Lists algorithm can be efficiently solved using a recursive or iterative approach. Let's explore a step-by-step breakdown of the iterative approach:
- Initialize a dummy node to serve as the head of the merged list.
- Initialize pointers (e.g., current and prev) to traverse the input lists and the merged list.
- Iterate through both input lists simultaneously:
- Compare the values of nodes at the current positions in both lists.
- Append the node with the smaller value to the merged list and move the corresponding pointer forward.
- Continue this process until reaching the end of either input list.
- Append the remaining nodes of the non-empty list to the merged list.
- Return the head of the merged list.
- Let's implement this algorithm in JavaScript:
const list1 = [2,3,8,12]
const list2 = [4,5,9,14]
const mergeSortedArrays = (array1,array2) => {
let index1 = 0;
let index2 = 0;
const newArray = []
while (index1 < array1.length || index2 < array2.length) {
if (array1[index1] < array2[index2]){
newArray.push(array1[index1])
index1++;
} else {
newArray.push(array2[index2])
index2++;
}
}
return newArray;
}
console.log(mergeSortedArrays(list1,list2));
Conclusion:
The Merge Two Sorted Lists algorithm, with its blend of simplicity and importance, holds significant relevance in programming and algorithmic problem-solving. By mastering its concepts, understanding implementation techniques, and exploring optimization strategies, developers can tackle a wide range of challenges related to sorting and data manipulation. So, whether you're merging sorted arrays or implementing merge sort, embrace the Merge Two Sorted Lists algorithm and let its power guide your journey through the world of JavaScript programming.