Longest Substring that contains no repeated Characters Algorithm
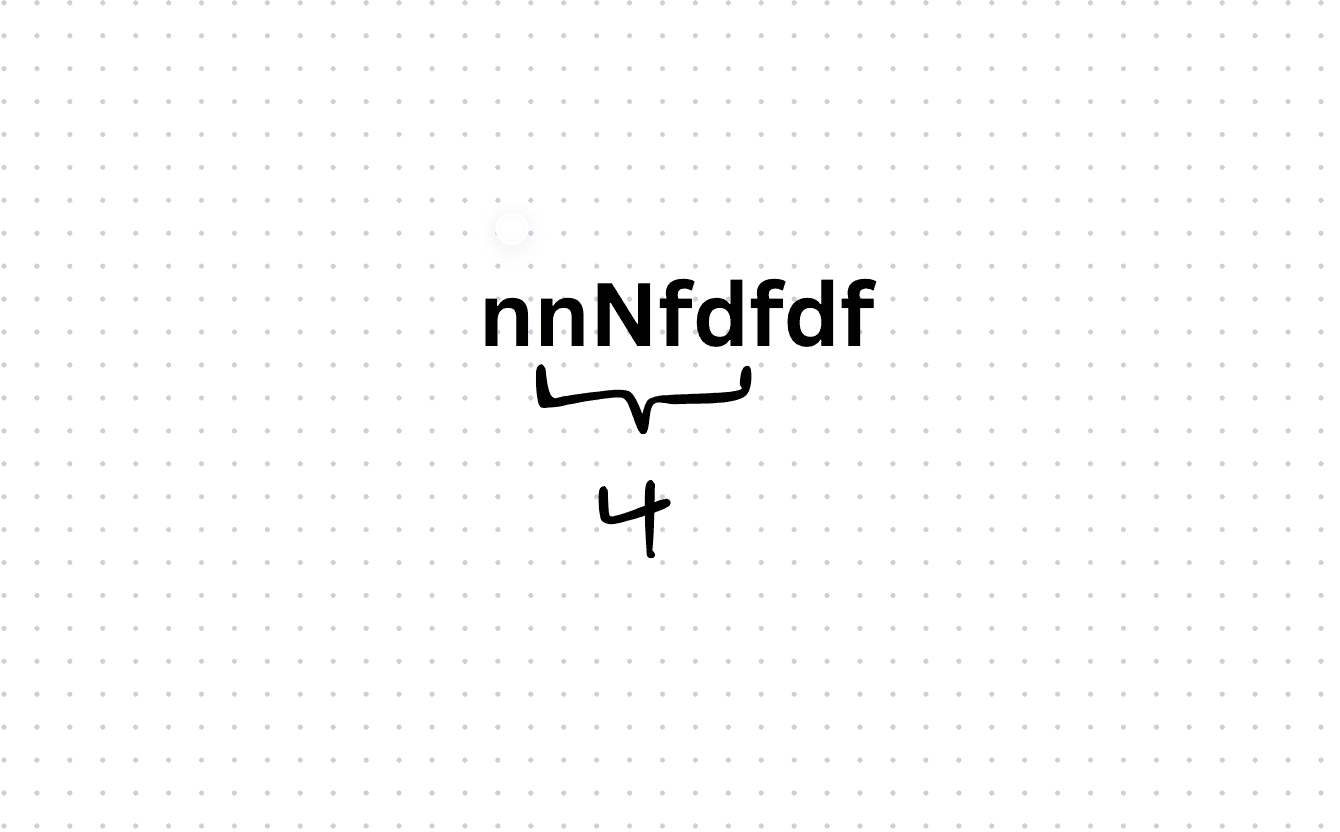
Given a string s, find the length of the longest substring that contains no repeated characters.
Example Input: "nnNfdfdf" Answer: 4
const solution = (s) => {
let left = 0;
let right = 1;
let max = 0;
while (right <= s.length) {
const substring = s.slice(left,right);
max = Math.max(substring.length, max)
const foundIndex = substring.indexOf(s[right])
if (foundIndex !== -1){
left += foundIndex+1;
right++;
} else {
right++;
}
}
return max
};
console.log(solution('nnNfdfdf'))