Text Highlighting Algorithm in TypeScript
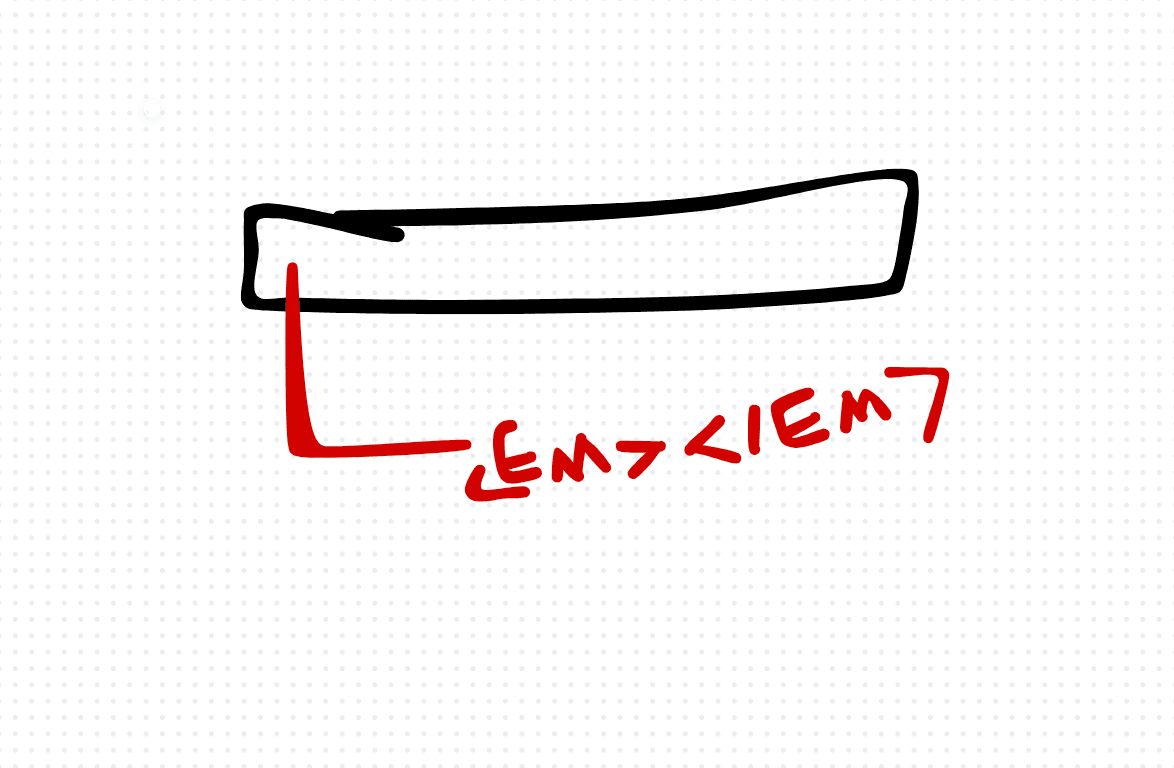
In modern web development, text highlighting is a common feature used in various applications, ranging from search engines to text editors. JavaScript provides a versatile platform for implementing such functionalities. In this article, we'll dissect a JavaScript function designed to highlight specific portions of a given string and explore how it can be optimized and expanded for broader use cases.
const highlight = (str, indexArray) => {
let returnStr = '';
let lastPoint = 0;
if (!Array.isArray(indexArray)) return str;
for (let i = 0; i < indexArray.length; i++) {
let start = indexArray[i][0];
const end = start + indexArray[i][1];
if (lastPoint < start) {
returnStr += `${str.slice(lastPoint, start)}`;
}
returnStr += `<em>${str.slice(start, end)}</em>`;
lastPoint = end;
}
if (lastPoint !== str.length) {
returnStr += `${str.slice(lastPoint, str.length)}`;
}
const regex = /<\/em><em>/gm;
returnStr = String(returnStr).trim().replace(regex, '');
return returnStr;
}
const res = highlight('asdasdassadsagrehetr',[[0,2],[3,4],[5,6]]);
console.log(res);
Analysis
The highlight function takes two parameters:
- str: The input string to be highlighted.
- indexArray: An array containing arrays of start and end indices for the portions of the string to be highlighted.
The function iterates over indexArray, slicing the input string and wrapping the specified substrings with <em> tags, indicating emphasis. It then removes redundant <em> tags that might occur consecutively. Finally, it returns the modified string.
Enhancements
While the provided function accomplishes its task, there are opportunities for enhancement and extension:
- Error Handling: The function currently assumes indexArray is always provided and of the correct format. Implementing error handling to handle edge cases would improve robustness.
- Performance Optimization: For large strings or numerous highlight segments, the current implementation might suffer from performance issues due to repeated string concatenation. Using an array to store substrings and joining them at the end can improve performance.
- Support for Custom Tagging: Instead of hardcoding the <em> tag, the function could accept an optional parameter for the tag to be used for highlighting, making it more flexible.
- Case Insensitivity: Adding support for case-insensitive matching could improve usability, especially for search-related applications.
- Whitespace Handling: Currently, whitespace within highlighted segments is preserved. Providing an option to trim or collapse whitespace within highlighted segments could be useful.
- Browser Compatibility: Ensuring cross-browser compatibility, especially for older browsers, might require additional polyfills or adjustments.
Conclusion
Text highlighting is a fundamental feature in many applications, and JavaScript offers a powerful platform for its implementation. By analyzing and enhancing existing functions like the one discussed here, developers can create more robust and versatile solutions that meet the evolving needs of modern web applications.