Implement a HashMap Algorithm
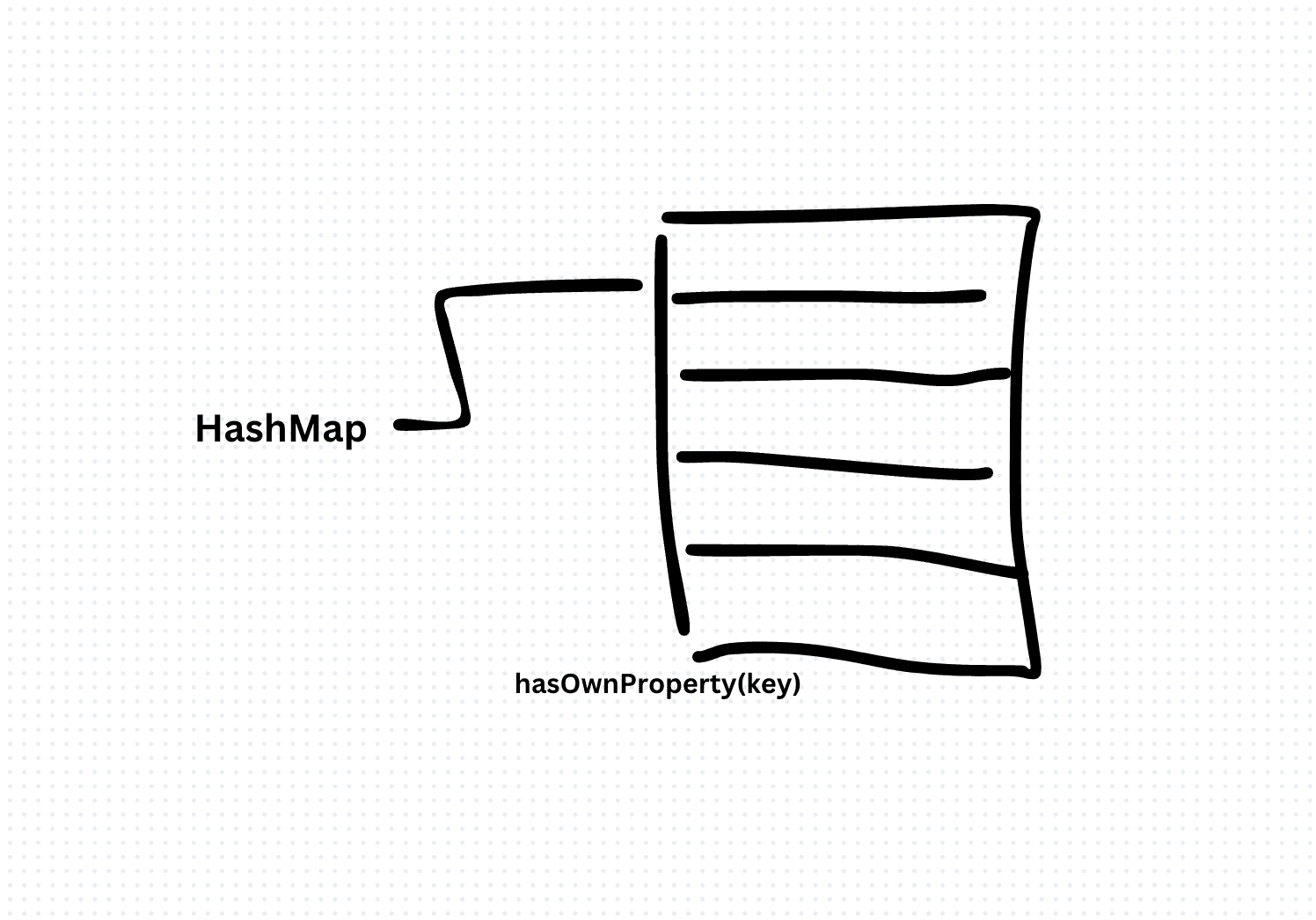
HashMaps are based on the concept of hashing, which involves converting a key into a hash code using a hash function. This hash code is then used to determine the index or position where the corresponding value should be stored in an array-like structure called a bucket. In case of hash collisions (i.e., two keys producing the same hash code), HashMaps typically resolve them using techniques like chaining or open addressing.
class HashMap {
constructor() {
this.map = {};
}
put(key, value) {
this.map[key] = value;
}
get(key) {
return this.map[key];
}
containsKey(key) {
return this.map.hasOwnProperty(key);
}
remove(key) {
if (this.map.hasOwnProperty(key)) {
delete this.map[key];
}
}
}
In this article, we've implemented a basic HashMap data structure in JavaScript. Although this implementation is simple and lacks some optimizations found in real-world HashMap implementations, it provides a solid foundation for understanding how HashMaps work under the hood. By further exploring HashMaps and experimenting with different techniques for handling collisions and resizing, developers can gain a deeper understanding of this versatile data structure and its applications in various programming scenarios.