Finding a Path in a 2D Array using TypeScript
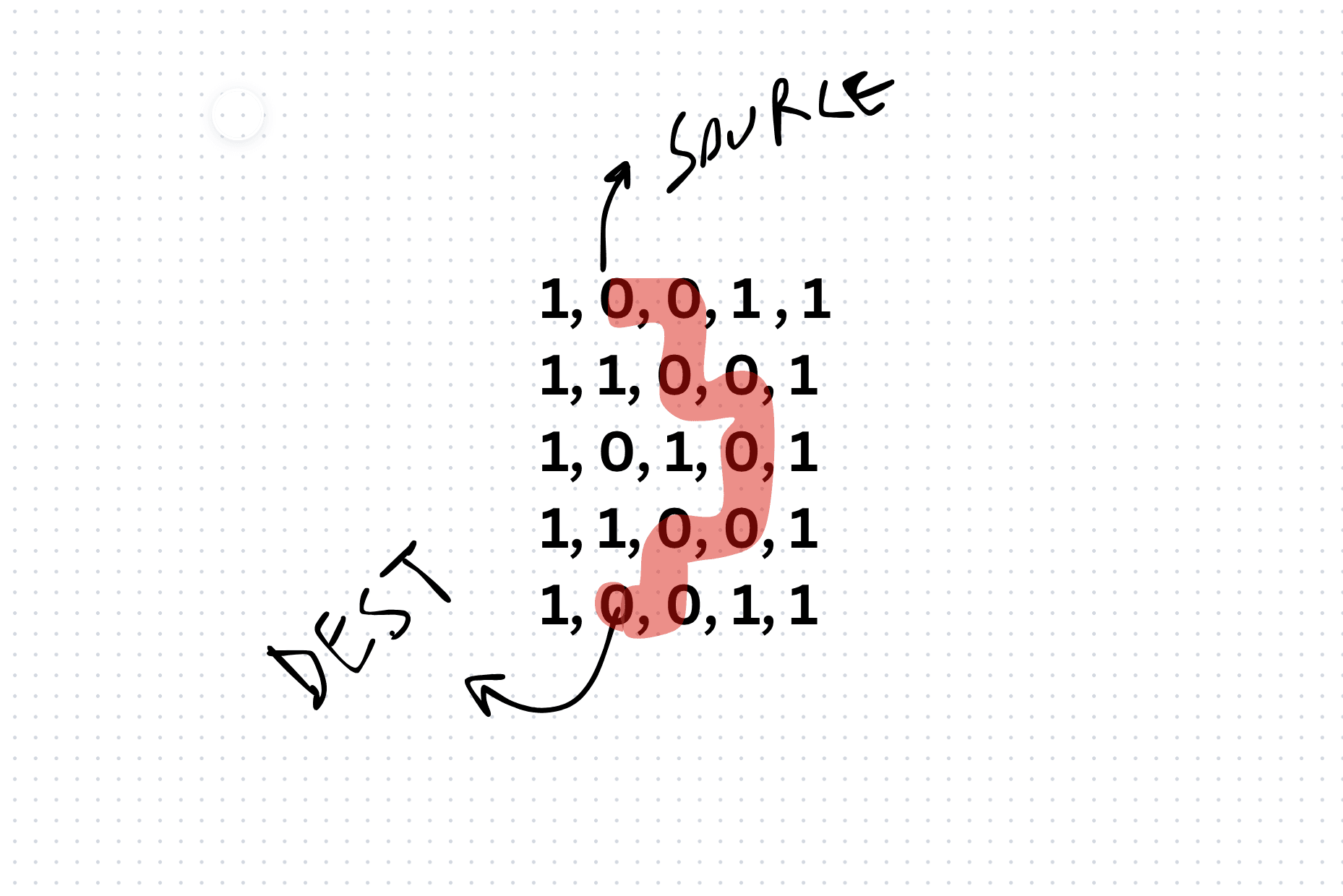
In this article, we'll explore a TypeScript algorithm that solves the problem of finding a path from a starting point to a destination point in a 2D array. The array contains 0s and 1s, where 0s represent empty spaces and 1s represent obstacles. The algorithm will navigate from the starting point to the destination point by moving up, down, left, or right between the empty spaces.
Given a 2D array arr of size m x n, where arr[i][j] is either 0 (empty space) or 1 (obstacle), and two coordinates startCol and startRow representing the starting point, and destCol and destRow representing the destination point. Find a path from the starting point to the destination point by moving up, down, left, or right between the empty spaces.
const checkIfItIsZero = (maze, x, y) => {
return x >= 0 && x < maze.length && y >= 0 && y < maze[0].length && maze[x][y] === 0;
};
const ifItIsNotReached = (reachedSoFar, x, y) => {
const checked = reachedSoFar.filter((item) => {
return item.x === x && item.y === y;
});
return checked.length === 0;
};
const solution = (maze, startRow, startCol, destRow, destCol) => {
const reachedSoFar = [{ x: startRow, y: startCol }];
const queue = [[startRow, startCol]];
while (queue.length > 0) {
const [x, y] = queue.shift();
if (x === destRow && y === destCol) {
return true;
}
const directions = [[-1, 0], [1, 0], [0, -1], [0, 1]];
for (const [dx, dy] of directions) {
const newX = x + dx;
const newY = y + dy;
if (checkIfItIsZero(maze, newX, newY) && ifItIsNotReached(reachedSoFar, newX, newY)) {
reachedSoFar.push({ x: newX, y: newY });
queue.push([newX, newY]);
}
}
}
return false;
};
console.log(solution(
[
[1, 0, 0, 1, 1],
[1, 1, 0, 1, 1],
[1, 0, 0, 1, 1],
[1, 0, 0, 1, 1],
[1, 0, 0, 1, 1],
],
0, 1, 4, 2
));