Fibonacci Sequence Algorithm
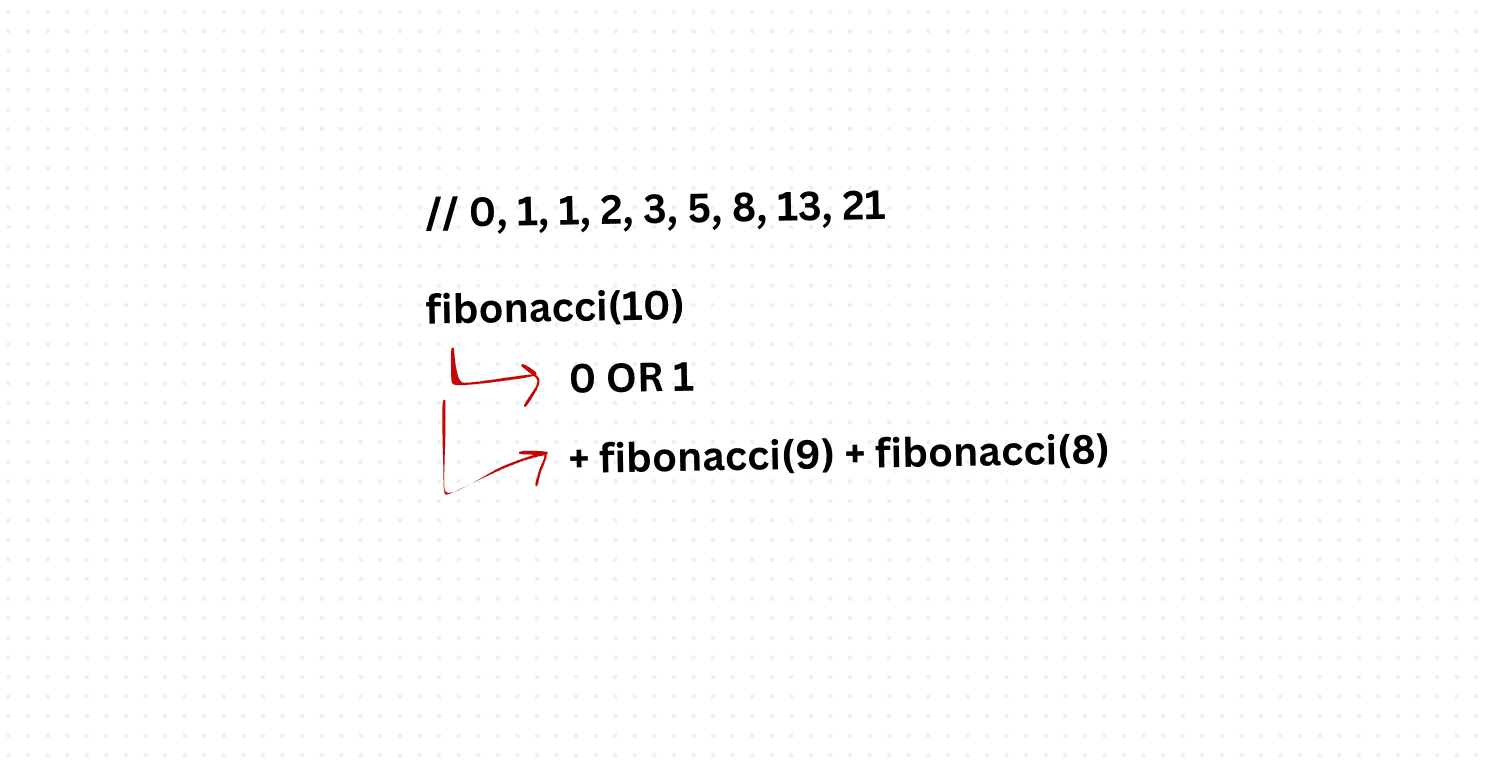
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. This sequence appears in various natural phenomena and has numerous applications in mathematics and computer science. In this article, we'll explore the Fibonacci sequence, understand its properties, and learn how to generate the nth Fibonacci number efficiently.
The sequence begins with 0 and 1, and each subsequent number is the sum of the two preceding numbers. The sequence starts as 0, 1, 1, 2, 3, 5, 8, 13, 21, and so on.
Generating the nth Fibonacci Number:
To generate the nth Fibonacci number efficiently, we can use either an iterative or a recursive approach. However, the recursive approach can be inefficient due to redundant calculations. Instead, we'll use an iterative approach that calculates each Fibonacci number in a loop.
Implementation in JavaScript:
// 0, 1, 1, 2, 3, 5, 8, 13, 21
const fibonacci = (n) => {
if (n <= 1) {
return n
} else if (n > 1) {
return fibonacci(n-1) + fibonacci(n-2)
}
}
console.log(fibonacci(10))
In this example, the fibonacciRecursive function is called with n = 10 to generate the 10th Fibonacci number. The result will be printed as "The 10th Fibonacci number is 55."
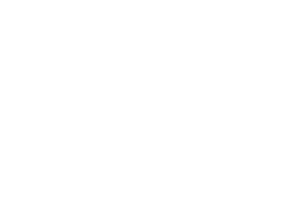
Conclusion:
The Fibonacci sequence, with its recursive definition, provides an intriguing concept in mathematics and computer science. While the recursive approach to generating Fibonacci numbers is elegant and intuitive, it may not be the most efficient for large values of n due to redundant calculations. Nonetheless, understanding the recursive nature of the Fibonacci sequence enhances our appreciation of its beauty and utility in various domains.